In almost every Arduino tutorial we’ve written we’ve used serial output for either printing text to terminal or plotting values. It is also invaluable as a debugging tool. We have rarely written about serial input, however, which is what this post is about.
- Things should be looking pretty familiar by now. The new stuff has to do with the serial monitor: In setup, look for Serial.begin(9600); which tells the Arduino to start communicating via text, at 9600 baud. The baud rate controls how much information is sent per second.
- Serial port library Arduino reference for hardware ports. Arduino Uno — hardware reference. Arduino Mega 2560 — hardware reference. Arduino Due — hardware reference. Projects, Articles and Tutorials. Using the Arduino serial port and serial monitor window. Getting serial input from the serial monitor window. Arduino serial thermometer.
- Don’t connect these pins directly to an RS232 serial port; they operate at +/- 12V and can damage your Arduino board. To use these extra serial ports to communicate with your personal computer, you will need an additional USB-to-serial adaptor, as they are not connected to the Mega’s USB-to-serial adaptor.
The final chunk of the loop function prints the current value to the Arduino Serial Monitor, and sends the current value to the digital feed on Adafruit IO. We also set last = current; so we can tell if the state of the button has changed in the next run of the loop. Aside from the digital input pins, the Arduino also boasts a number of analog input pins. Analog input pins take an analog signal and perform a 10-bit analog-to-digital (ADC) conversion to turn it into a number between 0 and 1023 (4.9mV steps). This type of input is good for reading resistive sensors.
What Can Serial Input Be Used for?
The possibilites with serial inputs are endless. Maybe you want to display text on an LCD display, punch in numbers to controll LEDs, control motor movement with arrow keys or send commands to decide which functions to call. No matter what you decide to use it for, your system reaches a higher level of interactivity.

How?
Using serial inputs is not much more complex than serial output. To send characters over serial from your computer to the Arduino just open the serial monitor and type something in the field next to the Send button. Press the Send button or the Enter key on your keyboard to send.
Coding wise, let’s dive into an example.
There are two important functions related to the serial input in the code above, and that is Serial.available()
and Serial.read()
.
Serial.available()
returns the number of characters (i.e. bytes of data) which have arrived in the serial buffer and that are ready to be read.
Serial.read()
returns the first (oldest) character in the buffer and removes that byte of data from the buffer. So when all the bytes of data are read and no new serial data have arrived, the buffer is empty and Serial.available()
will return 0.
Arduino Serial Input Basics Tutorial
If we send more than one character over serial with this code, the output will look like this:
But what if you want to send more than one character in handle it in a sensible way? No problemo!
Here we’ve introduced the readStringUntil()
function, which makes it possible to combine all the characters in the sent message into a single Arduino string. In this case we’re waiting for the n
character, which is the newline character that comes at the end of a string sent in the Arduino serial monitor.
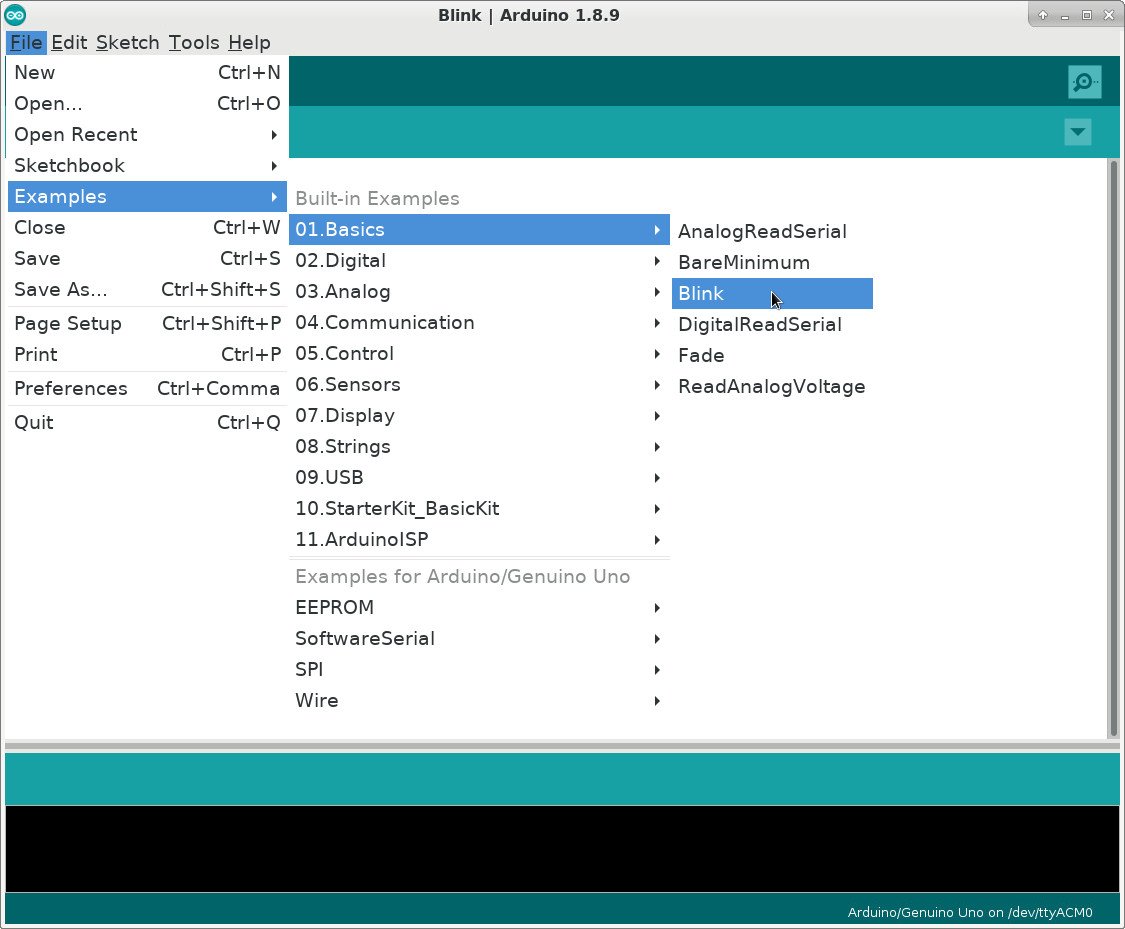
Sending Commands
A more usable scenario could be to send commands to the Arduino. Depending on what you send, the Arduino will perform different task. Here’s a somewhat abstract example on how to do this:
Notice the use of the equals()
function. This is a function in the Arduino String class which returns true
if the string in question is equal to the parameter string.
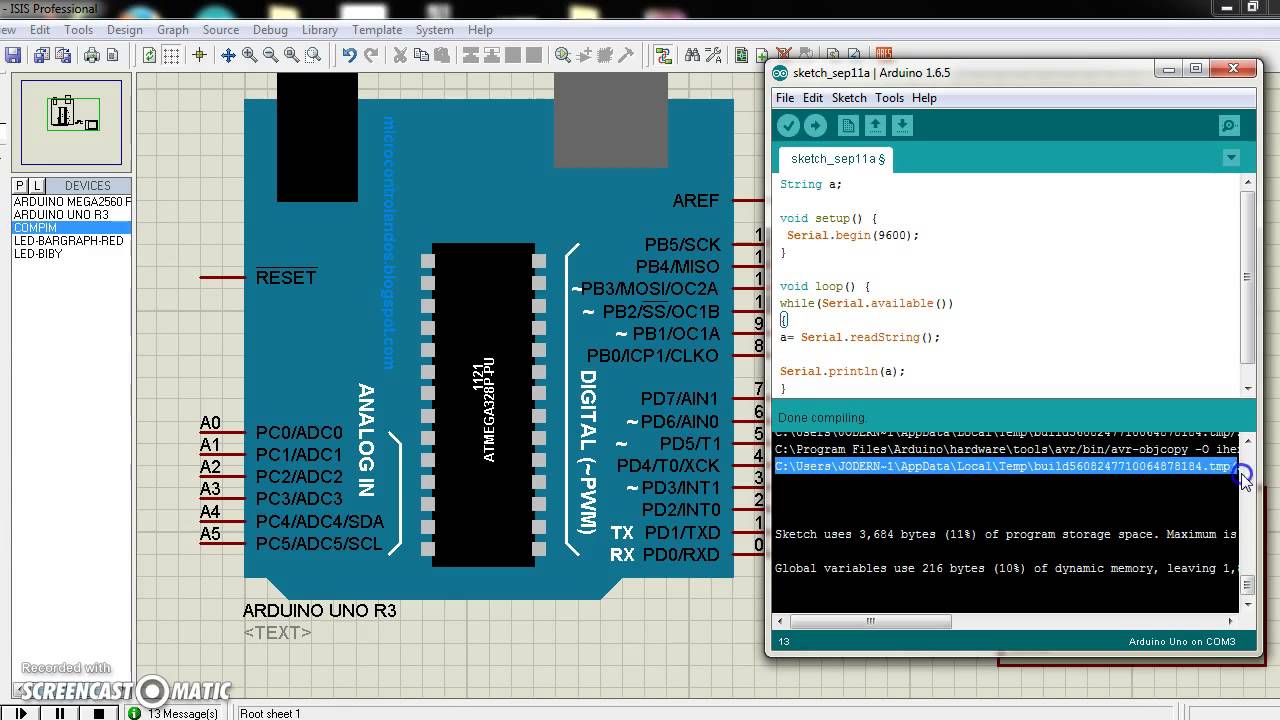
Summary
Arduino Serial Input Basics Keyboard
Serial inputs can be very useful in your Arduino project. It’s a bit more complex than serial output, but not by much! The key functions are Serial.available()
and Serial.read()
.
Take readings in real-time through the Arduino IDE's interface.
- 16,935 views
- 0 comments
- 13 respects
Components and supplies
| × | 1 | |
| × | 1 | |
| × | 1 | |
| × | 1 | |
| × | 1 |
Necessary tools and machines
About this project
Arduino Serial Input Basics Download
Let's learn to read data directly from the Arduino as text. We can see how it 'talks'!
Open up File > Examples > Basics > DigitalReadSerial, and set up your button the way we had it for the Button tutorial. Upload the sketch to your board.
CODE
Things should be looking pretty familiar by now. The new stuff has to do with the serial monitor:
In setup(), look for Serial.begin(9600);
which tells the Arduino to start communicating via text, at 9600 baud. The baud rate controls how much information is sent per second.
And in the loop(), you'll see the command Serial.println(buttonState);
– this tells the Arduino to take the current value of the button and print it to the monitor as a single line of text.
The monitor itself can be opened with the button at the top-right of the IDE:
Open it up, and you'll start to see a column of 0s. Push your button, and they will turn into 1s! Magic!
If you don't see the pretty 1s and 0s, check the settings at the bottom of the window:
- Autoscroll should be selected
- Newline should be selected
- 9600 baud should be selected (the rate at which the monitor expects to receive data)
Adapt This
What else can we do?
- Get real-time readings from a sensor
- Embed debug messages in code: Print to the serial monitor at crucial points in execution, to help with troubleshooting
Next Steps
Interact with this circuit in real-time, using Javascript! Arduino + NodeBots
See the whole series of Hackster 101 tutorials onHacksterandYouTube
Code
Schematics
Author
Alex Glow

- 18 projects
- 999 followers
Published on
July 18, 2016Members who respect this project
and 5 others
See similar projectsyou might like
Table of contents
